Java in industrial use on the sysWORXX CTR-700 controller
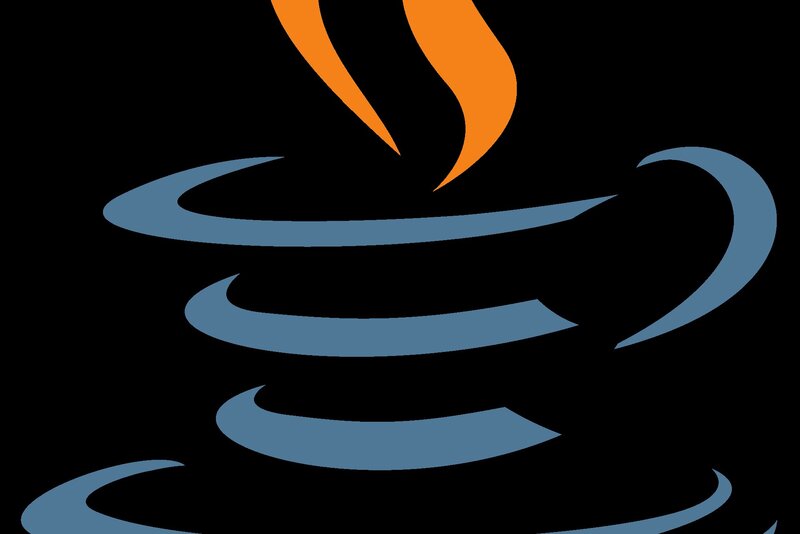
Application Description Java
General information
The object-oriented programming language Java has existed since 1995 and has always been one of the most popular and widely used. Java has constantly evolved over the years and is now used in programs and applications from a wide variety of industries. These include banks and credit institutions, for example, as well as insurance companies. Java is also frequently used in the field of web development, both for front- and back-end. Furthermore, Java can of course also be used for industrial applications, such as on the sysWORXX CTR-700.
Java is particularly characterized by its platform independence. Once created, Java programs can be executed on almost any hardware. It is irrelevant whether this is a smartphone, tablet, Windows or Linux PC. This independence requires only two things:
- The resources used must be available, for example communication interfaces or (digital or analog) inputs and outputs.
- A corresponding Java Runtime Environment (JRE) must be available on the executing system. For most operating systems this is always given
Another advantage of Java, which should not be underestimated, is the very wide distribution: every prospective software developer, computer scientist, or even hobby programmer will come into contact with Java in the early stages of his (professional) career. At many universities, for example, it is already treated in the first semesters. In the vastness of the internet Java is often recommended as "programming language for beginners".
Integration on the sysWORXX CTR-700
Due to the extensive platform independence of Java, the execution of a corresponding JRE is also possible on modern (automation) systems. With the Edge Controller sysWORXX CTR-700 the free Java implementation OpenJDK is offered by default. With this, Java programs can be easily executed and applied for industrial use.
A corresponding class library is available for the sysWORXX CTR-700, with which the various hardware interfaces and IOs can be integrated into a Java application. This means that Java can be used not only for generic data processing, but also for direct access to the hardware peripherals of the device.
Furthermore, a demo application illustrates how a Java application can be used in combination with the sysWORXX CTR-700.
MQTT demo including I/O connection
The following sample program is a Java application that is used as MQTT client on the sysWORXX CTR-700. This client connects to an MQTT broker, subscribes to given topics and switches the digital outputs of the device on or off according to the incoming messages.
The entire project can be downloaded at the end of this article and followed independently. The settings made in it are the same as those described in the following text.
Requirements
For this example, a Maven project must be created in Eclipse (4.11) and the corresponding dependencies must be imported. In addition to the necessary Maven dependencies (maven-dependency-plugin, maven-compiler-plugin, maven-jar-plugin), it is necessary for the MQTT client to include the"Eclipse Paho" project, as well as the"Java Native Access" library.
In addition, the driver class for the sysWORXX CTR-700 is required. This can be obtained from the virtual machine for the sysWORXX CTR-700 or taken from this demo project. The following figure shows how the project tree should look like.
In addition to the sysWORXX CTR-700 and a connection to a computer, an MQTT broker and a second controllable client are also required. For example, a mosquitto broker on the sysWORXX CTR-700 can serve as the MQTT broker, but any other broker to which a connection is possible can also be used.
The second MQTT client must be able to send messages to the specified topics to which the sysWORXX CTR-700 subscribes. For example, another Java application can be used for this. In this demonstration Node-RED is used for this purpose. The following figure shows the schematic flow:
Program flow
The structure and functionality of the main program can be found in the"Main.java"file. Before a connection can be established, all necessary variables are created and initializations are made.
It is necessary to create appropriate callback functions for the MQTT client for incoming messages and connection terminations. Incoming messages are not only accepted, but also output on the console. Disconnections are also displayed on the console.
When new messages arrive, the payload and the topic are each stored in an array so that they can be processed further outside the callback function. Likewise, a flag is set to signal a new message.
The main loop of the program is then executed. The client creates a connection with the specified IP address of an existing MQTT broker and subscribes to a total of four topics after a successful connection. The IP address used in the example is 192.168.64.100 and the default port for MQTT is 1883.
The following topics are used in the example:
- ctr700/do0
- ctr700/do1
- ctr700/do2
- ctr700/do3
After a successful connection is established, the program is in a continuous loop. This is only terminated when the connection to the MQTT broker is interrupted, whereupon it tries again to establish a connection, or the program is terminated (CTRL + C on the CTR-700). This loop only performs an evaluation when new MQTT messages arrive. Furthermore it writes in each cycle the digital inputs according to the digiOut array. This array holds the information about the circuit states of the digital outputs.
If the flag for a new incoming message has been set (see above), the following evaluation of the message is performed:
- An assignment is made to which subscribed topic the message matches.
- For the selected topic the content of the message is then evaluated:
- ON: The corresponding digital output is set to high (LED turns on). → digiOut[i] = true;
- OFF: The corresponding digital output is set to low (LED goes off). → digiOut[i] = false;
- If none of these apply, the message is ignored.
- Completion of the evaluation → exit from the program section.
The following table visualizes the program's responses to various topics and payloads.
Using the class library for the sysWORXX CTR-700
In order for the program flow described above to work and to use the hardware of the sysWORXX CTR-700, a corresponding instance of the CTR-700 I/O driver must be created and initialized. This is done at the beginning of the program, but can be at any point before the interface is used. This is done as follows:
Ctr700Drv ctr700 = new Ctr700Drv();
ctr700.init();
After initialization, the class for the sysWORXX CTR-700 can be used like any other class. For example, the following method is used for the later output of the digital outputs:
ctr700.setDigiOut(i, digiOut[i]);
Again the placeholderiserves for the used channels.
A documentation of each property and method of the class library, can be found in the Java file"Ctr700Drv.java".
The following is an excerpt from the demo. This shows the loop in which the program is permanently located after all required variables and classes have been initialized and a connection to the MQTT broker has been established.
Transfer and start of the application
In order to run the program on the CTR-700, it must first be compiled as an executable Jar file. In Eclipse, this can be done via the path"File → Export..."as shown in the following figure.
There the conversion to"Runnable JAR file"must be selected. In the next step, a"Launch configuration" must be selected, a path must be specified and the check mark for "Library handling" must be selected at"Package required libraries into generated JAR". The following figures show the respective steps.
After the conversion, the generated Jar file must be transferred to the device. The easiest way to do this is to use a SFTP tool like WinSCP.
In order to start the application now, the Linux console of the CTR-700 must be accessed. This can be done either via SSH or the serial service interface (µUSB). After logging in, it is necessary to change to the directory where the Jar file is located. Before it can be started, it is mandatory that the program gets rights to run. This can be done with the following command:
chmod +x demo.jar
After that the application can be started with the following command:
java -jar demo.jar
The following figure shows how this process could look like. For the access a Linux distribution was used. However, access under Windows is done in the same way.
Control via the second MQTT client
After running the application, the CTR-700 is ready to process data via MQTT. For example, control can be implemented very easily via Node-RED, which can also be used on the sysWORXX CTR-700 in parallel.
Injector nodes can be connected to an MQTT node for this purpose. The injectors then send a specific message including topics via the MQTT node to the broker, which forwards it to all subscribers. The following figures show how the injector messages can be configured and the Node-RED flow can be established:
There is one injector node each for switching the respective digital outputs on and off.
Concluding remarks
The considered example illustrates how easy the sysWORXX CTR-700 can be used with Java. Every Java developer can develop applications on this basis, which can interact directly with the interfaces of the device. Instead of this relatively simple application, sensors and actuators could then be connected to the digital inputs and outputs and controlled, for example.
For the start and the general use of the sysWORXX CTR-700 we offer a Linux VM for download on our product website. This includes a preconfigured Xubuntu installation. It also contains the class library which is required for software development with Java. There you can also find further information material, like the device manual, SD card image (operating system, etc.), but also further documentation for the sysWORXX CTR-700.