How our sysWORXX CTR-700 is programmed in C#
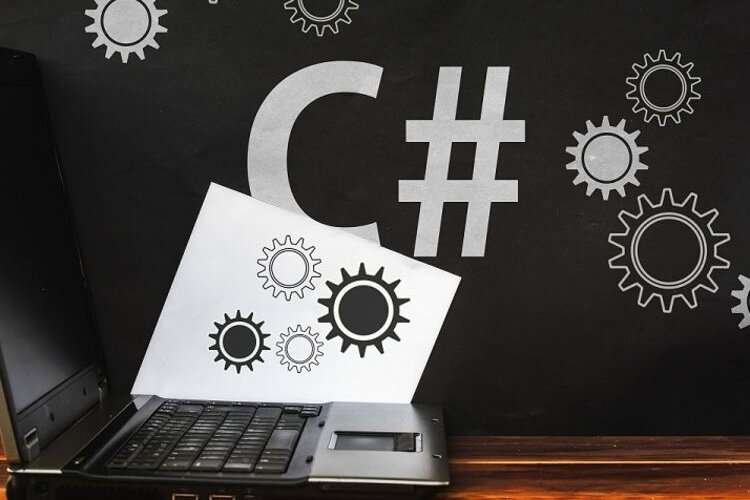
C# programming example
sysWORXX CTR-700 in C#
General Information
This Application Note describes in detail the programming of the sysWORXX CTR-700 in C# (pronounced as "C Sharp"). It thus extends the already published Application Notes for Java and Node-RED.
C# is a type-safe, object-oriented programming language whose origins lie in the family of C languages. It has a close relationship to C, C++ as well as Java and is therefore easy to use for programmers who already have experience with these languages. The most important design goal of the C# language was and is its platform independence. The first version of this language was released in 2001.
In terms of history, C# is closely linked to the .NET framework and is therefore also the most widely used on Windows platforms. Through projects such as Mono or .NET Core from Microsoft, applications written in C# can now also be run on other operating systems, such as Linux. In addition, Microsoft has announced that the next version of the .NET Framework (.NET 5) will include .NET Core and Mono and thus support an even greater number of target platforms at the same time.
The .NET Framework provides extensive class libraries, programming interfaces and utilities for application development. Likewise, many convenient tools are freely available, which enable easier use and allow seamless connection with other interfaces, such as connections to SQL servers, IIS, Office and many others. In addition, a runtime environment is provided for the execution of these applications.
Probably the best known development environment for C# programming is Visual Studio from Microsoft. This is offered both in a paid version, as well as in a free community version. According to Microsoft's licensing conditions, the community version may be used for non-commercial projects.
In addition, there are other development environments that are largely independent of Microsoft, such as Eclipse, MonoDevelop, JetBrains Rider and many others. Since C# with the .NET Framework contains a very extensive function package,
it can be used for the most diverse applications. C# is used, for example, to create Windows applications and services, but also for web applications, console programs and even video games.
Particularly in the IoT environment, C# has become widespread in recent years. Thus, there are now numerous, often open source software packages for the preparation of data as well as communication stacks for connecting edge controllers to higher-level cloud systems. With the runtime environment presented below, these software packages can also be used on the sysWORXX CTR-700.
How we work with C#
General information
A Linux distribution based on Debian is used as operating system for the sysWORXX CTR-700 edge controller. Suitable runtime environments for C# programs are provided by open source projects like Mono or .NET Core. These enable the execution of applications programmed in C# on embedded devices as well. In the case of the CTR-700, Mono is used. The manufacturer provides a corresponding class library for the CTR-700, with which the various hardware interfaces and IOs can be integrated and addressed in a C# application. Thus, it is possible not only to perform generic data processing with C#, but also to access the hardware of the device directly. Furthermore, the creation and commissioning of a C# application for the sysWORXX CTR-700 is illustrated by a demonstration.
Event-based control of the application
The following demo program shows a C# application that reads an analog input of the sysWORXX CTR-700 and outputs the measured value on the console. Additionally, it is possible to change the behavior of the program event-based via four buttons, which are connected to the digital inputs. The entire program can be downloaded and reproduced via the link provided at the end of the article.
Prerequisites
The community version of Visual Studio 2019 and the .NET Framework version 4.6.1 were used for this example. The use of alternative development environments is also possible, but if necessary, individual steps in the processing may differ slightly.
In Visual Studio, the following extensions
must also be installed via the NuGet package manager:
- For the use of mono functionalities, the extension"Mono.Posix"must be installed. This is required here for the used SIGINT interrupt.
- With older versions of Visual Studio it is possible that the class library of the CTR-700 cannot be translated. In this case the extension"Microsoft.Net.Compilers" must be installed. Optionally there is the possibility to install a Mono-Remote-Debugger.
This makes it possible to connect to the CTR-700 via a remote connection and then debug the program while it is running on the device. This function is supported in the described functionality only by Visual Studio. For this, addons such as the"MonoRemote Debugger "or"SMonoDebugger"are needed. Both offer a slightly different range of functions, but provide the same functionality at the core. Due to the somewhat easier handling, "VSMonoDebugger" is used for this example. The corresponding installation instructions can be found on the corresponding Marketplace page of Visual Studio, at the following link:
https://marketplace.visualstudio.com/items?itemName=GordianDotNet.VSMonoDebugger0d62
In addition, the class library provided by the manufacturer is required to access the IOs of the CTR-700. This can be taken from the demo project or the virtual machine for the CTR-700 (see download link SYS TEC electronic website). The class library must then be added to the C# project. In Visual Studio this is done by "Drag and Drop" or by right clicking on the project in the solution and"Add > Existing Element".
In addition to the sysWORXX CTR-700 and a connection to a Windows PC, four buttons are also required. These must be connected to the digital inputs 0 to 3. Later the behavior of the analog input can be controlled via these pushbuttons. Also a source for the generation of the analog values is needed. For example, a potentiometer, sensor, or a voltage source such as batteries or a laboratory power supply can be used. This is to be connected to the analog input 0. The following figure shows the schematic structure of the application:
Program flow
The flow and function of the main program can be found in the file "Program. cs". When the program is started, first all required variables are created and initializations are made. For the use of the CTR-700 object first an instance of the driver class must be created. Likewise, further auxiliary variables are created, for example for the state of the analog input (voltage or current measurement) and the associated conversion (digit and measured units), as well as the time interval of the measurement. Then the actual main loop (MainLoop) is called.
The MainLoop initializes as first interrupt events for the digital inputs 0 to 3 and the SIGINT interrupt for the termination of the program (key combination "Ctrl + C"). Likewise the associated interrupt handler for the DIs is registered. Then the program enters a while loop, which - typical for embedded devices - is executed until either the run switch is switched to the "STOP" position or the SIGINT interrupt is triggered. As long as the while loop is executed, a cyclic polling of the analog input AI0 is performed. By default, the applied voltage is measured every second and output to the console.
The output on the console requires the two auxiliary variables "digit" and "sMeassure". They are used to convert the measured analog value and to specify the appropriate physical unit. The value of one digit of the AD converter corresponds to 355.23
μV for voltage measurement or 819.87
nA for current measurement.
A separate event handler processes the inputs of the digital inputs via a switch case instruction. The following table shows which input, which property of the program changes:
Using the class function for the sysWORXX CTR-700
In order to execute the program flow described above and to use the hardware of the CTR-700, a corresponding instance of the CTR-700 I/O driver must be created. An additional initialization is not necessary.
var ctr700 = new Ctr700();
After that it is used like any other class in C#. For example, output to a digital output can be implemented:
ctr700.SetDigitalOutput(0, true);
This method sets digital output 0 to true (high = on). Digital and analog inputs can be read and used in a similar way. More extensive documentation can be found in the class library itself. The following program snippets show the main loop the program is in and the use of the event-based change of readout behavior:
MainLoop:
private static void MainLoop(Ctr700 ctr700)
{
UnixSignal sigint = new UnixSignal(Signum.SIGINT);
Here the initialization of the SIGINT interrupt takes place.
for (byte i = 0; i<4; i++)
{
ctr700.SetDigitalInputEvents(i, Ctr700InterruptTrigger.RisingEdge);
}
ctr700.DigitalInputChanged += Ctr700_DigitalInputChanged;
This for loop switches the event control of the DI's to the rising edge. When the loop is exited, they are released again.
while (ctr700.RunSwitch == true & !sigint.WaitOne(0))
{
Ctr700_SetAdc(ctr700, AdcMode);
Console.WriteLine("Measured Analog Input: " +
Math.Round(((ctr700.GetAnalogInput(bAnalogChannel) * digit ) /
1000000), 4) + " " + sMeassure);
System.Threading.Thread.Sleep(DelayThread);
}
Console.WriteLine("Shutting Down.");
The while loop forms the actual main program. It is executed as long as the run switch is "ON" and no SIGINT interrupt is triggered.
for (byte i = 0; i < 4; i++)
{
ctr700.SetDigitalInputEvents(i, Ctr700InterruptTrigger.None);
}
ctr700.DigitalInputChanged -= Ctr700_DigitalInputChanged;
}
If the main loop is exited, all DI events are deactivated again. This is analogous to the initialization above.
Event handler for the DIs:
private static void Ctr700_DigitalInputChanged(object sender,
DigitalInputChangedEventArgs args)
{
Console.WriteLine($"Digital input {args.Channel} is now
{(args.State ? "on" : "off")}");
switch(args.Channel)
{
case 0:
Console.WriteLine("Set Analog Input to current measurement.");
AdcMode = Ctr700AdcMode.Current;
break;
case 1:
Console.WriteLine("Set Analog Input to voltage measurement.");
AdcMode = Ctr700AdcMode.Voltage;
break;
case 2:
DelayThread = (DelayThread == 100) ?100 : DelayThread - 100;
Console.WriteLine("Speed-up the measurement interval by 100 ms.
Measurement is now at " + DelayThread + " ms");
break;
case 3:
DelayThread += 100;
Console.WriteLine("Slow-down the measurement interval by 100 ms.
Measurement is now at " + DelayThread + " ms");
break;
default:
DelayThread = 1000;
AdcMode = Ctr700AdcMode.Current;
break;
}
}
The event-based control consists in the of a switch instruction that changes the operation of the program depending on the digital input used.
Transferring, launching, and debugging the application
To run the program on the CTR-700, it must first be compiled and then transferred to the device. In Visual Studio, this is done using the"Build à Create Solution" function.
This creates an exe file, depending on the setting in the folder"bin/Debug" or"bin/Release", which must then be transferred to the CTR-700. Within Visual Studio this task is done transparently in the background by the Remote Plugin. However, the generated executable can also be loaded manually onto the CTR-700 using an SFTP tool such as WinSCP.
To start the application, the Linux console of the CTR-700 must be accessed. This is possible either via SSH, the service interface (μUSB), or, as described further below, the Mono Debugger plugin. After logging in, it is necessary to change to the directory where the exe file is located. The application will then be started with the following command:
mono DemoApplication.exe
Optionally, it is possible to start the exe file without the special mono call. However, to do this, it must be given the rights to run. This is done with the following commands:
chmod +x DemoApplication.exe
./DemoApplication.exe
In addition, the program can also be transferred via "VSMonoDebugger" and then debugged. Visual Studio establishes an SSH connection via this extension in order to transfer and start the program. First, however, the necessary SSH configuration must be made. This is done via the menu item"Extensionsà Monoà Settings". The following figure shows the configuration mask. The used IP address and login data have to be adapted according to the existing conditions.
The deployment and debug session is then executed via the menu "Extensions à Mono à Deploy and Debug (SSH)". In the output window of Visual Studio the occurring console outputs are then displayed. It is also possible to debug the program step by step.
As soon as the program is started, the voltage applied to the analog input is read out every second. Via the digital inputs (see table above) the measuring mode and also the measuring interval can be switched. Each change is output on the console.
Final notes
This example illustrates how easy it is to use the sysWORXX CTR-700 with C# and .NET. Any C# developer can use this as a basis to develop their own applications that interact directly with the interfaces of the device.
Instead of this simple application, which only reads in current and voltage, sensors could also be connected via the analog input, for example to obtain and evaluate environmental data.
For getting started and general use of the CTR-700, we offer a Linux VM for download on our product website. This includes a preconfigured Xubuntu installation. It also contains the class library, which is needed for software development with C#. Further information material, such as the device manual, SD card image (operating system, etc.) can also be found there, as well as additional documentation for the sysWORXX CTR-700.